authentication login and registration خطوات تنفيذ عمليات تسجيل الدخول والتسجيل في Laravel 9
Laravel 9 Authentication login and registration
install laravel 9 application
في البداية اذا لم يكن لارافيل مثبتا فعلينا تثبيتة عن طريق الامر الاتي
composer create-project laravel/laravel:^9.* auth
بعد تثبيت مشروع لارافيل تحت اسم auth
يجب الدخول علي فولدر المشروع بالامر التالي
cd auth
setup Database
بعد تثبيت تطبيق Laravel 9، نحتاج أولاً إلى إنشاء اتصال بقاعدة بيانات MySQL. لذلك، يتعين علينا فتح ملف .env وتحت هذا الملف، نحتاج إلى إضافة تكوين قاعدة بيانات MySQL مثل اسم قاعدة بيانات MySQL واسم مستخدم قاعدة بيانات MySQL وتفاصيل كلمة المرور. بمجرد تحديد هذه التفاصيل، سيتم إجراء اتصال بقاعدة بيانات MySQL في إطار عمل Laravel 9. يمكنك العثور أدناه على تفاصيل تكوين قاعدة بيانات MySQL.
env.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=auth
DB_USERNAME=root
DB_PASSWORD=
بعد ذلك التاكد من ان حقول جدول ال users بهذا الشكل
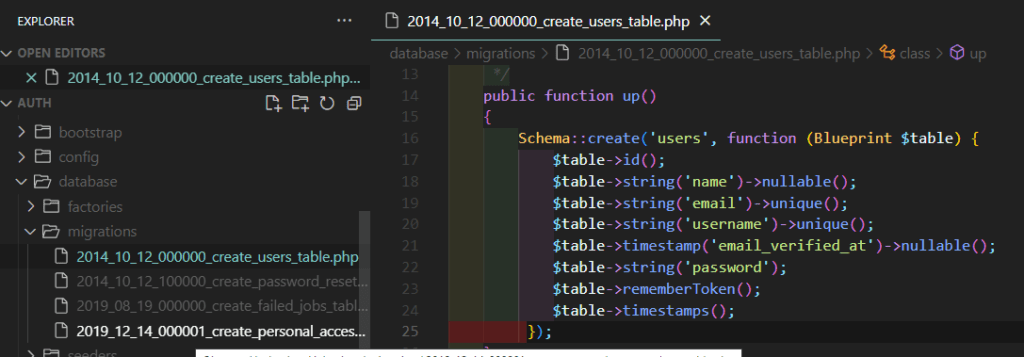
و الآن أصبحت جاهزة للترحيل، ما عليك سوى تشغيل الأمر التالي
php artisan migrate
ويتم الانشاء بهذا الشكل بعد الموافقة علي قاعدة بيانات جديدة باسم auth
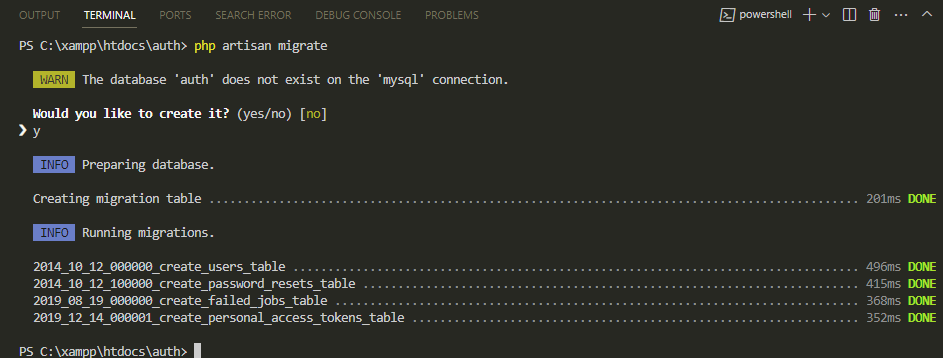
setup Model
لدينا بالفعل model باسم User
يمكن الوصول الية لو اتبعنا الرابط App\Models\User.php
كل ما علينا فعلة هو التاكد من الشكل التالي له
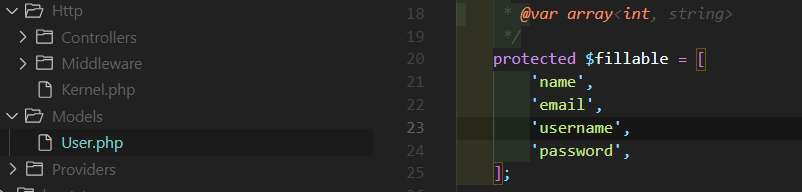
Setup Registration Controller
php artisan make:controller RegisterController
الآن، لقد قمت بالفعل بإنشاء RegistrerController والذي يمكنك العثور عليه لو اتبعت الرابط App\Http\Controllers\RegisterController.php الآن افتحه وتاكد من لصق الكود التالي أدناه:
validated());
auth()->login($user);
return redirect('/')->with('success', "Account successfully registered.");
}
}
Setup Registration Request
php artisan make:request RegisterRequest
الآن، لقد قمت بالفعل بإنشاء RegisterRequest والذي يمكنك العثور عليه لو اتبعت الرابط App\Http\Requests\RegisterRequest.php الآن افتحه وتاكد من لصق الكود التالي أدناه:
'required|email:rfc,dns|unique:users,email',
'username' => 'required|unique:users,username',
'password' => 'required|min:8',
'password_confirmation' => 'required|same:password'
];
}
}
Setup Login Controller
php artisan make:controller LoginController
الآن، لقد قمت بالفعل بإنشاء LoginController والذي يمكنك العثور عليه لو اتبعت الرابط App\Http\Controllers\LoginController.php الآن افتحه وتاكد من لصق الكود التالي أدناه:
getCredentials();
if(!Auth::validate($credentials)):
return redirect()->to('login')
->withErrors(trans('auth.failed'));
endif;
$user = Auth::getProvider()->retrieveByCredentials($credentials);
Auth::login($user);
return $this->authenticated($request, $user);
}
/**
* Handle response after user authenticated
*
* @param Request $request
* @param Auth $user
*
* @return \Illuminate\Http\Response
*/
protected function authenticated(Request $request, $user)
{
return redirect()->intended();
}
}
Setup Login Request
php artisan make:request LoginRequest
الآن، لقد قمت بالفعل بإنشاء LoginRequest والذي يمكنك العثور عليه لو اتبعت الرابط App\Http\Requests\LoginRequest.php الآن افتحه وتاكد من لصق الكود التالي أدناه:
'required',
'password' => 'required'
];
}
/**
* Get the needed authorization credentials from the request.
*
* @return array
* @throws \Illuminate\Contracts\Container\BindingResolutionException
*/
public function getCredentials()
{
// The form field for providing username or password
// have name of "username", however, in order to support
// logging users in with both (username and email)
// we have to check if user has entered one or another
$username = $this->get('username');
if ($this->isEmail($username)) {
return [
'email' => $username,
'password' => $this->get('password')
];
}
return $this->only('username', 'password');
}
/**
* Validate if provided parameter is valid email.
*
* @param $param
* @return bool
* @throws \Illuminate\Contracts\Container\BindingResolutionException
*/
private function isEmail($param)
{
$factory = $this->container->make(ValidationFactory::class);
return ! $factory->make(
['username' => $param],
['username' => 'email']
)->fails();
}
}
Setup Logout Controller
php artisan make:controller LogoutController
الآن، لقد قمت بالفعل بإنشاء LogoutController والذي يمكنك العثور عليه لو اتبعت الرابط App\Http\Controllers\LogoutController.php الآن افتحه وتاكد من لصق الكود التالي أدناه:
Setup Home Controller
php artisan make:controller HomeController
الآن، لقد قمت بالفعل بإنشاء HomeController والذي يمكنك العثور عليه لو اتبعت الرابط App\Http\Controllers\HomeController.php الآن افتحه وتاكد من لصق الكود التالي أدناه:
Setup Routes
بعد ذلك، سنقوم بإعداد مساراتنا التي يمكننا العثور عليها عند اتباع الرابط routes/web.php حيث أننا قمنا بالفعل بإعداد وحدات التحكم والمدققين للمشروع
'App\Http\Controllers'], function()
{
/**
* Home Routes
*/
Route::get('/', 'HomeController@index')->name('home.index');
Route::group(['middleware' => ['guest']], function() {
/**
* Register Routes
*/
Route::get('/register', 'RegisterController@show')->name('register.show');
Route::post('/register', 'RegisterController@register')->name('register.perform');
/**
* Login Routes
*/
Route::get('/login', 'LoginController@show')->name('login.show');
Route::post('/login', 'LoginController@login')->name('login.perform');
});
Route::group(['middleware' => ['auth']], function() {
/**
* Logout Routes
*/
Route::get('/logout', 'LogoutController@perform')->name('logout.perform');
});
});
لانشاء صفحات العرض يجب في البداية إنشاء مجلد (layouts) داخل المجلد /resources/views وملف auth-master.blade.php بداخلة ليصبح المسار الناتج هو resources/views/layouts/auth-master.blade.php
auth-master.blade.php
Signin Template · Bootstrap v5.1
@yield('content')
ايضا داخل مجلد (layouts) داخل المجلد /resources/views ننشيء ملف app-master.blade.php بداخلة ليصبح المسار الناتج هو
resources/views/layouts/app-master.blade.php
app-master.blade.php
Fixed top navbar example · Bootstrap v5.1
@include('layouts.partials.navbar')
@yield('content')
ثم ننشيء مجلد (partials) داخل المجلد resources/views/layouts و بداخلة ننشيء ملف ليصبح المسار الناتج هو
resources/views/layouts/partials/navbar.blade.php
navbar.blade.php
بعد ذلك ننشيء ملف جديد ليصبح المسار الناتج هو
resources/views/layouts/partials/messages.blade.php
messages.blade.php
@if(isset ($errors) && count($errors) > 0)
@foreach($errors->all() as $error)
- {{ $error }}
@endforeach
@endif
@if(Session::get('success', false))
@if (is_array($data))
@foreach ($data as $msg)
{{ $msg }}
@endforeach
@else
{{ $data }}
@endif
@endif
ثم ننشيء مجلد (auth) داخل المجلد resources/views و بداخلة ننشيء ملف ليصبح المسار الناتج هو
resources/views/auth/register.blade.php
register.blade.php
@extends('layouts.auth-master')
@section('content')
@endsection
ثم ننشيء ملف ليصبح المسار الناتج هو
resources/views/auth/login.blade.php
login.blade.php
@extends('layouts.auth-master')
@section('content')
@endsection
ثم ننشيء مجلد (partials )داخل مجلد (auth) داخل المجلد resources/views و بداخلة ننشيء ملف ليصبح المسار الناتج هو
resources/views/auth/partials/copy.blade.php
copy.blade.php
© {{date('Y')}}
ثم ننشيء مجلد (home ) داخل المجلد resources/views و بداخلة ننشيء ملف ليصبح المسار الناتج هو
resources/views/home/index.blade.php
index.blade.php
@extends('layouts.app-master')
@section('content')
@auth
Dashboard
Only authenticated users can access this section.
View more tutorials here »
@endauth
@guest
Homepage
Your viewing the home page. Please login to view the restricted data.
@endguest
@endsection
bootstrap
ثم نقوم بتنزيل ملفات bootstrap ولصقها في فولدر public/bootstrap
ووضع الروابط التالية في ملف app-master , auth-master
finish
ثم أعد تشغيل خادم التطبيق الخاص بك باستخدام الكود أدناه:
php artisan serve
تفضل بزيارة http://127.0.0.1:8000 على متصفحك لعرض مدونتك الجديدة.
Sharing to