CRUD هو اختصار لـ “Create, Read, Update, Delete” وهو نمط شائع في تطوير البرمجيات يستخدم في إنشاء وقراءة وتحديث وحذف البيانات في قواعد البيانات. Laravel هو إطار عمل PHP قوي وشائع الاستخدام و يمكنك معرفة المزيد عن عمليات ادارة البيانات
في Laravel، يمكنك بناء عمليات CRUD بسهولة باستخدام Eloquent ORM ومكتباته المدمجة. يمكنك استخدام الـ migrations لإنشاء جداول قاعدة البيانات، والـ models للتفاعل مع تلك الجداول، والـ controllers لإدارة العمليات المختلفة مثل الإضافة والقراءة والتحديث والحذف، وأخيرًا تستخدم الـ views لعرض البيانات.
يوجد في Laravel أيضًا ميزات مفيدة مثل توجيه الطلبات (Routing) والتحقق (Authentication) والتراكب (Middleware) التي تسهل عملية بناء تطبيقات CRUD بشكل أكثر فعالية وسلاسة.
جدول المحتويات
1 - download & install laravel 9
في البداية نقوم بانشاء مشروع جديد laravel 9 وذلك عن طريق استخدام برنامح visual studio code او command prompt وادخال الكود :
composer create-project laravel/laravel:^9.0 crud
بمجرد تشغيل هذا الأمر، سيتم إنشاء دليل crud وتحت هذا الدليل سيتم تنزيل تطبيق Laravel 9 وتثبيته.
cd crud
2 - setup database connection
بعد تثبيت تطبيق Laravel 9، نحتاج أولاً إلى إنشاء اتصال بقاعدة بيانات MySQL. لذلك، يتعين علينا فتح ملف .env وتحت هذا الملف، نحتاج إلى إضافة تكوين قاعدة بيانات MySQL مثل اسم قاعدة بيانات MySQL واسم مستخدم قاعدة بيانات MySQL وتفاصيل كلمة المرور. بمجرد تحديد هذه التفاصيل، سيتم إجراء اتصال بقاعدة بيانات MySQL في إطار عمل Laravel 9. يمكنك العثور أدناه على تفاصيل تكوين قاعدة بيانات MySQL.
env.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=crud
DB_USERNAME=root
DB_PASSWORD=
3 – Create Post Model ,Controller & Migration For CRUD App
ضمن هذه الخطوة، يتعين علينا وضع جدول المنشورات ضمن قاعدة بيانات MySQL من تطبيق Laravel 9 و controller , model لذلك يتعين علينا تشغيل الأمر التالي في موجه الأوامر.
php artisan make:model post -mcr
بذلك قد انشأنا migration, controller and model باسم post
بعد تشغيل الأمر أعلاه، ستجد ملفًا جديدًا ضمن دليل قاعدة البيانات/الترحيلات. لذلك يتعين علينا فتح هذا الملف وتحت هذا الملف، يتعين علينا تحديد التعليمات البرمجية التالية ضمن ملف الترحيل هذا لإنشاء جدول المنشورات في قاعدة بيانات MySQL.
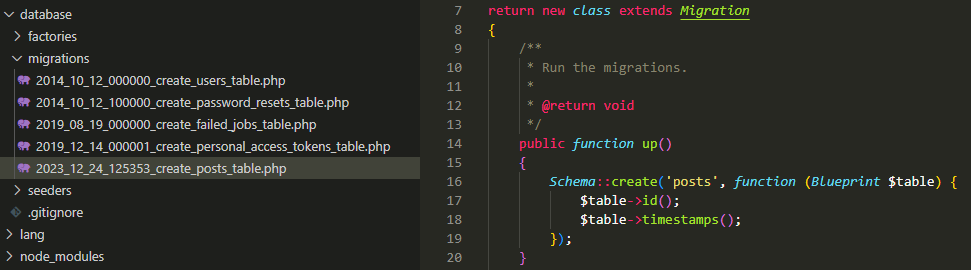
id();
$table->string('title');
$table->text('body');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('posts');
}
};
بذلك قد انشأنا جدول في قاعدة بيانات MYSQL يسمي posts بة id,title,body and timestamps
بعد الكود أعلاه في ملف الترحيل، الآن لإنشاء جدول في قاعدة بيانات MySQL من تطبيق Laravel 9، لذلك، يتعين علينا تشغيل الأمر التالي في موجه الأوامر وسوف يتم ترحيل تعريف جدول MySQL إلى قاعدة بيانات MySQL وإنشاء جدول الطلاب في قاعدة بيانات MySQL من تطبيق Laravel 9 هذا.
php artisan migrate
بذلك قد انشأنا جدول في قاعدة بيانات MYSQL يسمي posts يتكون من :
id, title, body and timestamps
وتكون النتيجة كالتالي
4 - Create Functions in controller
وبعد إنشاء وحدة تحكم CRUD باسم ملف PostController.php ضمن دليل app/Http/Controllers.
بعد ذلك، قم بإنشاء الوظائف التي تقوم بتخزين البيانات وفهرستها وتحديثها وتدميرها وإنشائها وعرضها وتحريرها.
يمكنك إضافتها إلى الملف app/Http/Controller/PostController.php الموضح أدناه.
store function
اذهب الي store function وأضف الكود التالي داخل الأقواس المتعرجة الفارغة:
$request->validate([
'title' => 'required|max:255',
'body' => 'required',
]);
Post::create($request->all());
return redirect()->route('posts.index')
->with('success','Post created successfully.');
index function
اذهب الي index function لجلب جميع المنشورات من قاعدة البيانات وترسل البيانات إلى صفحة Posts.index. وأضف الكود التالي داخل الأقواس المتعرجة الفارغة:
public function index()
{
$posts = post::all();
return view('posts.index', compact('posts'));
}
all function
وفي النهاية يجب أن يبدو ملف PostController.php بالشكل التالي:
validate([
'title' => 'required|max:255',
'body' => 'required',
]);
Post::create($request->all());
return redirect()->route('posts.index')
->with('success', 'Post created successfully.');
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$request->validate([
'title' => 'required|max:255',
'body' => 'required',
]);
$post = Post::find($id);
$post->update($request->all());
return redirect()->route('posts.index')
->with('success', 'Post updated successfully.');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$post = Post::find($id);
$post->delete();
return redirect()->route('posts.index')
->with('success', 'Post deleted successfully');
}
// routes functions
/**
* Show the form for creating a new post.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('posts.create');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$post = Post::find($id);
return view('posts.show', compact('post'));
}
/**
* Show the form for editing the specified post.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$post = Post::find($id);
return view('posts.edit', compact('post'));
}
}
5 - Model
بعد إنشاء ملف Post.php داخل المجلد App/Models.
أضف الكود التالي داخل فئة النشر وأسفل استخدام HasFactory
protected $fillable = [
'title',
'body',
];
6 - Add Routes
بعد إنشاء وظائف وحدة التحكم (controller) ونموذج النشر(model)، يجب عليك إضافة مسارات لوظائف وحدة التحكم الخاصة بك.
افتح المسارات/web.php واحذف المسار المعياري الذي أنشأه التطبيق. استبدله بالكود أدناه لتوصيل وظائف وحدة التحكم بالمسارات الخاصة بها:
// returns the home page with all posts
Route::get('/', PostController::class .'@index')->name('posts.index');
// returns the form for adding a post
Route::get('/posts/create', PostController::class . '@create')->name('posts.create');
// adds a post to the database
Route::post('/posts', PostController::class .'@store')->name('posts.store');
// returns a page that shows a full post
Route::get('/posts/{post}', PostController::class .'@show')->name('posts.show');
// returns the form for editing a post
Route::get('/posts/{post}/edit', PostController::class .'@edit')->name('posts.edit');
// updates a post
Route::put('/posts/{post}', PostController::class .'@update')->name('posts.update');
// deletes a post
Route::delete('/posts/{post}', PostController::class .'@destroy')->name('posts.destroy');
لتوصيل المسارات، افتح الراوت (route) واضف app/Http/Controllers/PostController.php
وأضف السطر أدناه تحت السطر
use Illuminate\Support\Facades\Route;
بالشكل الاتي
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PostController;
وفي النهاية يجب أن يبدو ملف Route.php بالشكل التالي:
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PostController;
// returns the home page with all posts
Route::get('/', PostController::class .'@index')->name('posts.index');
// returns the form for adding a post
Route::get('/posts/create', PostController::class . '@create')->name('posts.create');
// adds a post to the database
Route::post('/posts', PostController::class .'@store')->name('posts.store');
// returns a page that shows a full post
Route::get('/posts/{post}', PostController::class .'@show')->name('posts.show');
// returns the form for editing a post
Route::get('/posts/{post}/edit', PostController::class .'@edit')->name('posts.edit');
// updates a post
Route::put('/posts/{post}', PostController::class .'@update')->name('posts.update');
// deletes a post
Route::delete('/posts/{post}', PostController::class .'@destroy')->name('posts.destroy');
7 - blade view
index.balde.php
بعد انشاء ملف Index.blade.php يكون محتواه كالاتي :
Posts
table posts
title
Body
Edit
Delete
@foreach($posts as $post)
{{ $post->title }}
{{ $post->body }}
Edit
@endforeach
create.balde.php
بعد انشاء ملف create.blade.php يكون محتواه كالاتي :
Create Post
edit.balde.php
بعد انشاء ملف edit.blade.php يكون محتواه كالاتي :
Edit Post
finish
ثم أعد تشغيل خادم التطبيق الخاص بك باستخدام الكود أدناه:
php artisan serve
تفضل بزيارة http://127.0.0.1:8000 على متصفحك لعرض مدونتك الجديدة.
Sharing to
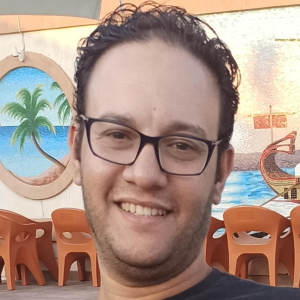